Dynamically Adding Ports with the PortPairExtender
Sometimes it's useful to add ports that simply pass data through the operator without changing it - 'through' ports. If your operator does not need input and output ports, best practice is to add through ports to control the process execution order. ThePortPairExtender, a sub-class ofPortExtender, ensures that the through ports are pair-wise.
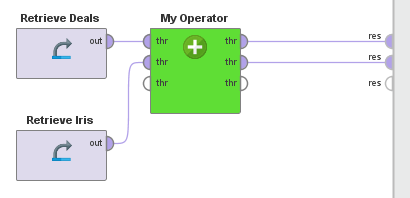
ThePortPairExtenderis also used to pass input data from a super operator to its subprocesses. You can find more details in the advanced chapter aboutsuper operators.
Adding through ports
To define through ports, simply add aPortPairExtenderas a private variable, using the following code:
private final PortPairExtender dummyPorts = new DummyPortPairExtender("through", getInputPorts(), getOutputPorts());
Then, initialize the ports and add the pass through rule to handle the meta data:
dummyPorts.start(); getTransformer().addRule(dummyPorts.makePassThroughRule());
Add the command to pass the data from the input through ports to the output through ports at the end of thedoWork()
method.
dummyPorts.passDataThrough();
Simple example operator with through ports
public class MyOwnOperator extends Operator { private PortPairExtender dummyPorts = new DummyPortPairExtender("through", getInputPorts(), getOutputPorts()); public MyOwnOperator(OperatorDescription description) { super(description); dummyPorts.start(); getTransformer().addRule(dummyPorts.makePassThroughRule()); } @Override public void doWork() throws OperatorException { LogService.getRoot().log(Level.INFO, "Doing something..."); // PASS THROUGH PORTS dummyPorts.passDataThrough(); } }
The next step is toadd parametersto your operators.